Tracking Envoyer Releases in Sentry
Note: this article I wrote originally appeared on the Humaan blog.
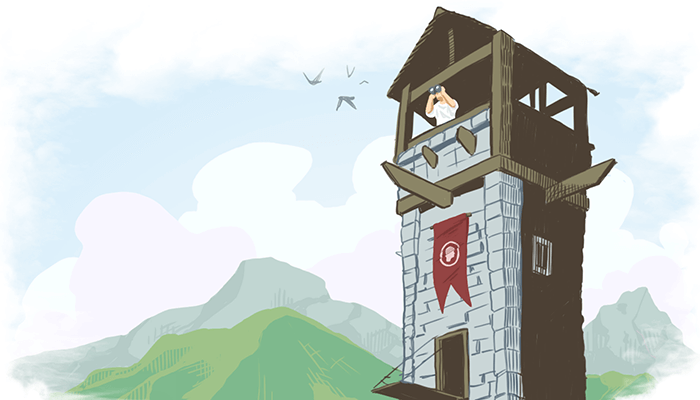
Tracking down bugs can be hard. Damn hard. And figuring out how or when they cropped up in the first place can be even harder. Recently, we launched our social media aggregator platform, Waaffle. It’s written in Laravel and deployed with Envoyer, but we also took the opportunity to try out Sentry for tracking bugs.
Spoiler alert: it was awesome.
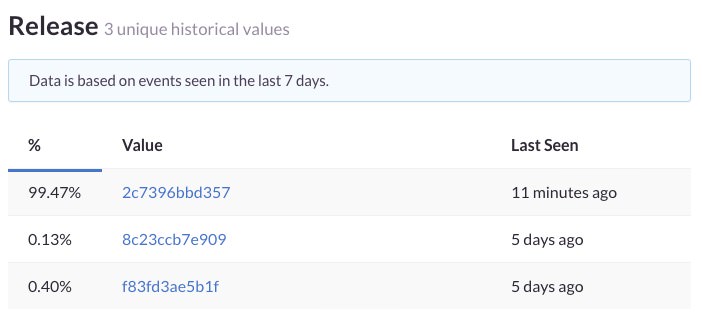
One of the features I really like is the ability to track releases, which means you can tell at a glance which release was responsible for your bug. We’ve got a deployment hook in Envoyer that pings Sentry when we deploy a new release. Our hook runs in the “After” section of the “Activate New Release” action and contains the following command:
curl https://sentry.io/api/hooks/release/builtin/project_id/project_webhook_token/ \
-X POST -H 'Content-Type: application/json' -d '{"version": "{{ sha }}"}'
(FYI, it’s the very last post-deployment hook we run, just in case another hook fails.)
The Sentry PHP package includes a section in the config for specifying your app version. However, things can get tricky if, like us, you’re using, say, git commit hashes instead of version numbers.
Thankfully, Sentry’s Laravel package provides code for a nice release hash you can use for getting your git commit hash:
'release' => trim(exec('git log --pretty="%h" -n1 HEAD')),
Which if you run in Artisan’s tinker mode, gives you something like this:
1a2b3c
Now the problem: we use Envoyer for our deployments, which downloads the tarball of a specified branch/release/tag instead the entire git repository. That tarball doesn’t contain any of the git history in it, so it’s not technically a repository, thus running the Sentry Laravel command won’t give you a commit hash. If you try, you’ll get the following lovely error:
fatal: Not a git repository (or any of the parent directories): .git
Which means if Sentry tracks an error and saves it, you’ll see there’s no release attached to the issue. Drats!
Somehow, we need a way to get the current commit hash from our Envoyer deployments so we can tell Sentry how to get the current commit. Thankfully, Envoyer comes to the rescue!
In the Envoyer docs, under Deployment Hooks, you’ll see a section on how you can get the current git commit hash (or sha1 hash). Now, this hash is actually the first 12 characters of the current git commit hash - aka. something you can use in the deployment lifecycle.
From here, we want to target the current release directory, so let’s create a deployment hook in the “After” section of “Activate New Release” and call it “Write Git Hash to File” with the user “forge”:
echo "{{ sha }}" > {{release}}/.commit_hash
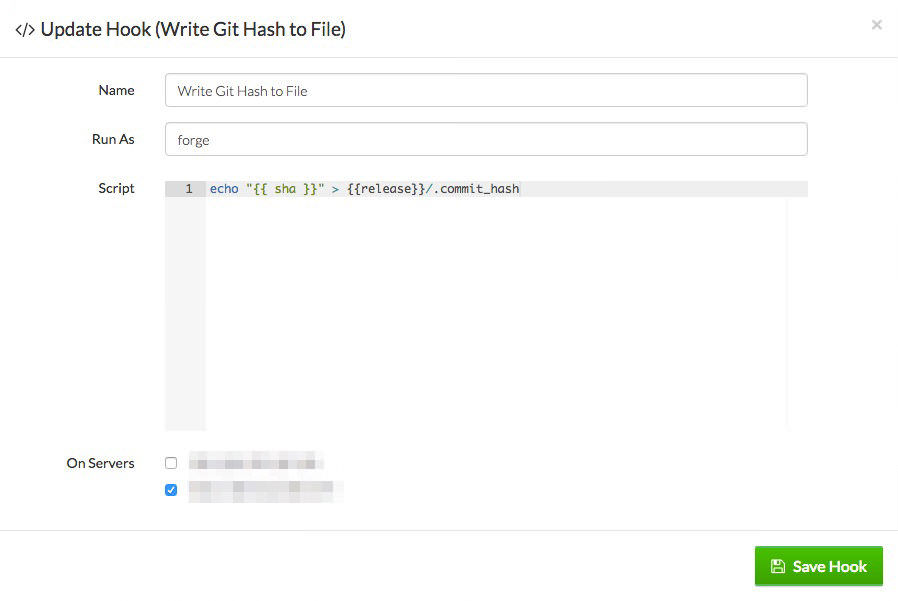
Pretty simple, huh? All it does is echoes the current git hash to stdout
(standard output), then we redirect that output to a file called .commit_hash
in the current release directory. The single arrow (>
) means “set the file .commit_hash
to 0
length, then append to it”. If we used two arrows (>>
) we’d only append to the file, which we wouldn’t want to do - though technically it doesn’t really matter given the release directory has just been created, but it’s the principle of the thing!
Once we’ve got that file written on every deployment, we need to tell Sentry how to get that commit hash! If you wanted to, you could change the config so Sentry fetches the release, like so:
'release' => trim(exec('echo "$(< .commit_hash)"')),
(Note that I’m using echo and redirection here, I don’t want to get into the UUOC debate!)
Except this only works if you have that .commit_hash
file in every environment, which we don’t. So, we’re stuck between a rock and a hard place. What we need is a solution that uses the .commit_hash
file if it exists, or falls back to a git repository, or finally, returns null
if neither of those exist.
In our project, we’ve got a small helpers file with all functions that exist in the global namespace. That’s where we put our solution:
<?php | |
if (!function_exists('get_commit_hash')) { | |
/** | |
* Checks to see if we have a .commit_hash file or .git repo and return the hash if we do. | |
* | |
* @return null|string | |
*/ | |
function get_commit_hash() | |
{ | |
$commitHash = base_path('.commit_hash'); | |
if (file_exists($commitHash)) { | |
// See if we have the .commit_hash file | |
return trim(exec(sprintf('cat %s', $commitHash))); | |
} elseif (is_dir(base_path('.git'))) { | |
// Do we have a .git repo? | |
return trim(exec('git log --pretty="%h" -n1 HEAD')); | |
} else { | |
// ¯\_(ツ)_/¯ | |
return null; | |
} | |
} | |
} |
Pretty basic really - just an if/elseif/else block with three possible outcomes. Note that I’m using the base_path Laravel helper, so we can be sure we’ve got the full path to the file/folder in question. Then, in our Sentry configuration file we have the following for the release:
'release' => get_commit_hash(),
Now whenever Sentry needs the commit hash, it’ll call that function and get its return value. Nice! And for those of you concerned about the overheads of getting the commit hash, you can do a php artisan config:cache
so the hash is only calculated once. I highly recommend caching the app configuration as part of your deployment process to help to make things that little bit faster. That file is located in bootstrap/cache/config.php
and you can verify the existence of this cached value by doing the following (in the root of the project, with the configuration cached):
grep -n --context=3 \'release\' bootstrap/cache/config.php
You should see something like this:
484- 'sentry' =>
485- array (
486- 'dsn' => 'https://xxxxx:xxxxx@sentry.io/project_id',
487: 'release' => '1a2b3c4d5e6f',
488- ),
489- 'auth' =>
490- array (
(The line numbers to the left indicate where in the file it was found.)
Now you’re set! The next time you do a deployment, you’ll have Sentry correctly logging issues with the current release throughout the life of the project.
Happy tracking, folks!